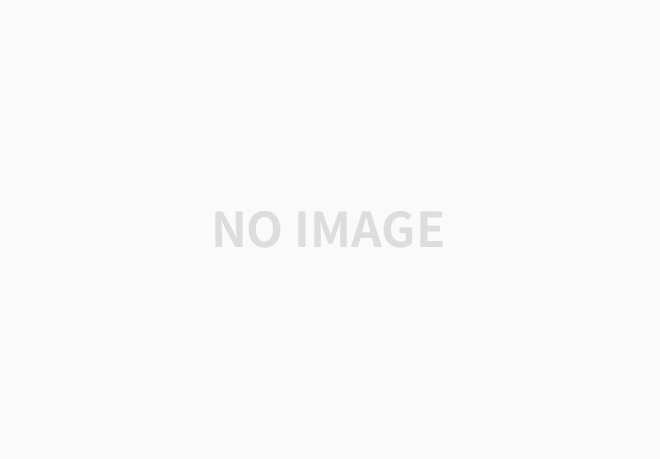
Palindrome Number - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
Given an integer x, return true if x is palindrome integer.
An integer is a palindrome when it reads the same backward as forward.
- For example, 121 is a palindrome while 123 is not.
Example 1:
Input: x = 121
Output: true
Explanation: 121 reads as 121 from left to right and from right to left.
Example 2:
Input: x = -121
Output: false
Explanation: From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
Example 3:
Input: x = 10
Output: false
Explanation: Reads 01 from right to left. Therefore it is not a palindrome.
Constraints:
- -231 <= x <= 231 - 1
Follow up: Could you solve it without converting the integer to a string?
Solution1: string revert comparision
문자열로 처리하는 코드가 가장 간단한 방법입니다.
class Solution:
def isPalindrome(self, x: int) -> bool:
x = str(x)
return x == x[::-1]
Solution2: full revert integer comparision
10으로 나누면서 다른 변수에 값을 복제하는 방식입니다.
컴퓨터에서 문자열보다 숫자를 처리하는게 빠릅니다.
문자열 하나는 최소 1바이트 이상의 값 전체를 비교하는 작업으로
일반적으로 숫자 타입을 연산하는 명령어가 CPU를 더 적게 사용합니다.
class Solution:
def isPalindrome(self, x: int) -> bool:
if x < 0 : return False
revert = 0
tmp = x
while tmp:
revert = revert*10 + tmp%10
tmp //= 10
return x == revert
Solution3(best): half revert integer comparision
Palindrome을 확인하기 위해 완전한 반전을 할 필요는 없습니다.
절반만 반전해봐서 서로 같은지 확인하면 됩니다.
다만 자릿수가 홀수인 경우 처리를 위해 코드가 약간 더 복잡해집니다.
최종적으로 2번 방법의 절반 수준의 loop를 돌아 결과를 확인합니다.
class Solution:
def isPalindrome(self, x: int) -> bool:
if x < 0 or (x > 0 and x % 10 == 0): return False
revert_half = 0
while x > revert_half:
revert_half = revert_half * 10 + x % 10
x = x//10
return x == revert_half or x == revert_half//10
GitHub - junyeollee/CODINGTEST_PRACTICE
Contribute to junyeollee/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Computer Science > CODINGTEST_PRACTICE' 카테고리의 다른 글
[LeetCode] 14. Longest Common Prefix (python3) (0) | 2022.07.12 |
---|---|
[LeetCode] 13. Roman to Integer (python3) (0) | 2022.07.08 |
[LeetCode] 1. Two Sum (python3) (0) | 2022.07.03 |
[LeetCode] 975. Odd Even Jump (python3) (0) | 2022.07.03 |
[LeetCode] 929. Unique Email Addresses (python3) (0) | 2022.06.23 |