반응형
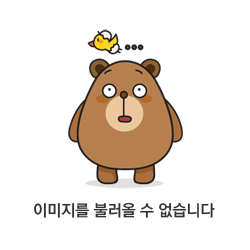
https://leetcode.com/problems/longest-common-prefix/
Longest Common Prefix - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
Write a function to find the longest common prefix string amongst an array of strings.
If there is no common prefix, return an empty string "".
Example 1:
Input: strs = ["flower","flow","flight"]
Output: "fl"
Example 2:
Input: strs = ["dog","racecar","car"]
Output: ""
Explanation: There is no common prefix among the input strings.
Constraints:
- 1 <= strs.length <= 200
- 0 <= strs[i].length <= 200
- strs[i] consists of only lowercase English letters.
Solution: O(nlogn)
- sort array
- just compare first string and last string to get LCP
class Solution:
def longestCommonPrefix(self, strs: List[str]) -> str:
if len(strs) == 1: return strs[0]
strs.sort()
if strs[0] == "": return ""
prefix = ""
for i, char in enumerate(strs[0]):
if char == strs[-1][i]:
prefix += char
else:
break
return prefix
Solition2: O(n)
작성중
반응형
'Computer Science > CODINGTEST_PRACTICE' 카테고리의 다른 글
[LeetCode] 151. Reverse Words in a String 기록 (0) | 2022.11.13 |
---|---|
[LeetCode] 20. Valid Parentheses 기록 (2) | 2022.11.12 |
[LeetCode] 13. Roman to Integer (python3) (0) | 2022.07.08 |
[LeetCode] 9. Palindrome Number (python3) (0) | 2022.07.06 |
[LeetCode] 1. Two Sum (python3) (0) | 2022.07.03 |